Armor Slot Ids Minecraft
- Armor Slot Ids Minecraft 1.8
- Armor Slot Ids Minecraft
- Armor Slot Id Minecraft
- Armor Slot Ids Minecraft Bedrock
- basic knowledge of how data packs work
MINECRAFT ARMOR STAND. Enter the text id of an item (for example stone or ironsword) into the appropriate slots. You can enter an arbitrary item for the head. All slot index ranges are inclusive and reflect the indices observed in the Minecraft protocol. For the window properties (additional data in each window, e.g. Smelting progress or enchantments), refer to the table in the Window Property packet. Using -1 as slot index and as window id will set the cursor item (the stack dragged with the mouse). Armor is a special type of item which players and certain types of mobs can wear for protection, decreasing the damage inflicted upon them. 1 Mechanics 2 List of armor (by slot) 2.1 Helmets/Caps 2.2 Chestplates/tunics 2.3 Leggings/pants 2.4 Boots 3 Armor types 3.1 Leather armor 3.2 Golden armor 3.3 Chainmail armor 3.4 Iron armor 3.5 Diamond armor 3.6 Netherite armor 3.7 Elytra 3.8 Turtle shell.
- custom crafting recipes
- custom crafting benches
- floor crafting
- Armor, replace 'minecraft' with your mod's name and replace 'diamondhelmet' into your armor id, for example, rubymod:rubyhelmet Step 4: Duplicate the previous command for 3 times but replace the 'slot.armor.head' into 'slot.armor.chest' or 'slot.armor.legs' and finally, 'slot.armor.feet' in those new duplicated procedure blocks Step 5: Done.
- The Inventory is the pop-up menu that a player uses to manage items they carry. From this screen, a player can equip armor, craft items on a 2x2 grid, and equip tools, blocks, and items on the Hotbar displayed at the bottom of the screen. A player's Minecraft skin is displayed here as well. 1 Overview 2 Creative mode inventory 3 Trivia 4 Video The inventory is opened and closed by pressing the.
custom crafting recipes are basically .json files which you place inside your data pack to add new recipes to the game. You can either write the code manually or use an online recipe generator (like this one https://crafting.thedestruc7i0n.ca).
once you obtain a recipe .json file, you need to place it in: your_datapack > data > namespace > recipes
the code below is a .json file generated with the online recipe generator which contains information about our custom crafting recipe. I will try my best to explain what every line does.
let's start with the first line. the 'type' tag specifies what type of recipe this is. The 2 types are:
- craftine_shaped - the items must match the given pattern
- crafting_shapeless - the items can be placed in any order
the next tag is 'key', this section is used to tell the game what item type every symbol represents. In this example, the '#' represents a diamond and the 'G' represents a golden apple.
the 'result' tag is used to specify the output item of the recipe
the 'group' tag is used to sort all your recipes, this can be any name. All recipes that have the same group name will be displayed together in the recipe book (screenshot included)
so every recipe with the group name 'newRecipes' will be displayed in a group window in the recipe book like shown in the screenshot.
the other type of crafting is 'shapeless' crafting which looks like this:
the only difference is that this recipe uses 'ingredients' instead of 'pattern'. It works the same way as shaped crafting but the items can be placed on the grid in any order.
However, this method comes with a big limitation which is no NBT support. This means you can only craft regular items with no additional data.
Armor Slot Ids Minecraft 1.8
Custom crafting benchesUnlike custom crafting recipes, this method allows you to include NBT in your recipes but isn't as nice as the custom crafting recipe method.
For this method, you only need 1 line of code so you can paste it in a command block or in your function file if you're using a data pack.
Also, I'm using an armor stand with a tag 'crafter' as a reference point for the crafting bench but you can use coordinates if you want.
here is the code:
this command is testing if the block at the armor stands position is a dropper with a specific formation of items and if so, it overwrites the droppers inventory, clearing out all the items all and places an output item in the droppers inventory. In this case, I'm crafting a stick with a custom name if there are 2 diamonds in a stick shape (4b and 7b).
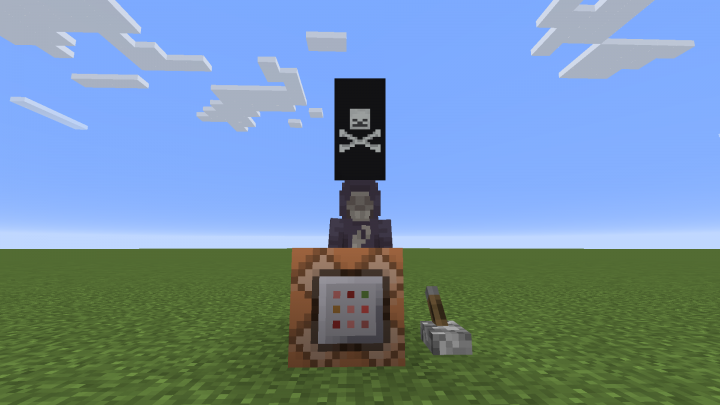
Here's the layout of the slots. for example 'Slot:4b' would be the middle square
There's just one more thing to do and that is to implement a crafting limitation.
what I mean by that is add some code so you can't craft another stick if a stick from the previous crafting is already inside the dropper.
the piece of code you need to add is: unless block ~ ~ ~ dropper{Items:[{Slot:4b,id:'minecraft:stick',Count:1}]}
this prevents the user from crafting another item if there's already an item inside the crafting grid. In this example, my crafting recipe will output a stick in slot 4b so that's why I'm testing if there is no stick in slot 4b.
so the code with the limitation implemented will look like this:
Floor crafting
floor crafting is pretty common for command block creations and even data packs. Its basically throwing 2 or more items on the ground and combining them into a new item.
the basic syntax is as follows:
Armor Slot Ids Minecraft
execute from item #1 > execute to item #2 > summon output item > remove item #1 and #2so the commands would look like this: